SDK UI & Core for iOS
Overview
Introduction
Mobile SDK UI & Core for iOS is a software development kit with open-source code that can be used to integrate iOS applications with the ecommpay payment platform.
SDK UI & Core for iOS provides the functionality for interaction of customers with the user interface and for interaction of a mobile application with the payment platform which allows sending and receiving necessary information during payment processing. Additionally, the open-source code available with SDK UI & Core for iOS provides flexibility for configuring the user interface in accordance with the aspects of the application.
SDK UI & Core for iOS can be embedded in mobile applications developed for iOS version 15.6 or later. The libraries and code examples are available on GitHub. To access, use the following URLs:
- List of SDK UI & Core for iOS releases: https://github.com/ITECOMMPAY/mobile-sdk-ios-ui/releases
- Code examples: https://github.com/ITECOMMPAY/mobile-sdk-ios-ui/tree/master/IntegrationSamples
This article describes how to work with SDK UI & Core for iOS and provides code examples in Swift and Objective-C.
Capabilities
The following functional capabilities are supported by SDK UI & Core for iOS:
- Processing different types of payments made with cards and Apple Pay as well as other payment methods available for the merchant's project. Supported payment types include:
- One-time one-step purchases.
- One-time two-step purchases (an authorisation hold can be placed via the SDK and subsequent debiting of the authorised amount is carried out via Gate or Dashboard).
- COF purchases (they can be registered via the SDK and then managed via Gate or Dashboard).
Note: In case of card and Apple Pay payments, the payment interface described in this article is used. With other payment methods, Payment Page is used during payment processing. - Performing payment card verification (it involves debiting a zero amount from the customer's card).
- Checking current payment information.
- Auxiliary procedures and additional capabilities to boost payment acceptance rates:
- Submission of additional payment information.
- Payment retries.
- Cascade payment processing.
- Collecting customer data.
- Additional capabilities to improve user experience:
- Saving customer payment data.
- Payment interface language support.
- Sending email notifications with the list of purchased items to customers.
- Customising the appearance of the payment interface including the colour scheme settings and the option to add the logo.
Workflow
Generally, the following workflow is relevant when purchases are processed with the use of SDK UI & Core for iOS.
- In the user interface of a mobile application, the customer initiates a purchase by clicking the payment button or in a different fashion set up on the merchant side.
- In the mobile application, a set of parameters for creating a payment session is generated. Then, with the help of SDK UI & Core for iOS, this set is converted into a string for signing, and the string is sent to the server side of the merchant web service.
- On the server side of the merchant web service, the parameters can be checked and supplemented if necessary, and the signature to the final parameter set is generated, following which the prepared data is sent back to SDK UI & Core for iOS.
- With the help of SDK UI & Core for iOS, a payment session is initiated in the payment platform.
- On the payment platform side, the payment interface is prepared in accordance with the invocation parameters, and the data for opening the interface is passed to the customer's device.
- In the mobile application, the payment form is displayed to the customer.
- The customer selects a payment method (if no method was selected when the payment session was initiated), specifies the necessary information, and confirms the purchase.
- SDK UI & Core for iOS sends a purchase request to the payment platform.
- On the payment platform side, the payment is registered and all necessary technical actions are performed; these actions include sending the required data to the payment environment—to the providers and payment systems.
- The payment is processed in the payment environment. Then the payment result information is received in the payment platform.
- In the payment platform, the information about the payment result is processed and a callback is sent to the server side of the web service.
- The information about the purchase result is sent from the payment platform to SDK UI & Core for iOS.
- The notification with the result information is displayed to the customer in the user interface.
Interface
When card and Apple Pay payments are processed, the customer interacts with the user interface designed by the ecommpay specialists. This user interface can be customised: you can change its colour and add your company's logo.
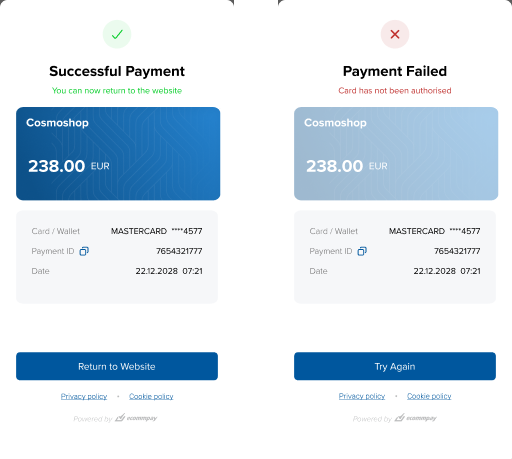
Setup
Integration steps
To integrate the web service with the ecommpay payment platform by using SDK UI & Core for iOS:
- Address the following organisational issues of interaction with ecommpay:
- If your company has not obtained a project identifier and a secret key for interacting with ecommpay, submit the application for connecting to the ecommpay payment platform.
- If your company has obtained a project identifier and a secret key for interacting with ecommpay, inform the technical support specialists about the company's intention to integrate by using SDK UI & Core for iOS and coordinate the procedure of testing and launching the functionality.
- Complete the following preliminary technical steps:
- Download and link the SDK UI & Core for iOS libraries.
- Ensure the collection of data necessary for opening the payment form. The minimum data set needed in order to open the payment form consists of the project, payment, and customer identifiers as well as of the payment amount and currency.
- Ensure signature generation for the data on the server side of the mobile application.
- Ensure the receipt of and the response to the notifications from SDK UI & Core for iOS as well as the receipt of and the response to the callbacks from the payment platform on the web service side.
- With the technical support specialists, coordinate the timeline and the main steps of integrating, testing (including testing available payment methods), and launching the solution.
- For testing, use the test project identifier and the details of test cards.
- For switching to the production mode, change the value of the test project identifier to the value of the production project identifier received from ecommpay.
If you have any questions about working with SDK UI & Core for iOS, contact the ecommpay technical support specialists (support@ecommpay.com).
Importing libraries in Swift
To add a library into your iOS app:
- Copy the
ecommpaySDK.xcframework
file in the project folder of you iOS app. - Add the library into your project. When using Xcode 12, you need to do the following:
- Open the target of your project.
- Select .
- Click +.
- Click Add Other.
- Select the
ecommpaySDK.xcframework
file and click Add.
- Add key NSCameraUsageDescription with value
permission is needed in order to scan card
to the Info.plist file. - If your iOS app does not use user location information, add the NSLocationWhenInUseUsageDescription key with the
fraud prevention
value in the Info.plist file.The ecommpay libraries code does not request user location if the request is not initiated by the host app, but the App Store requires that the NSLocationWhenInUseUsageDescription key value is not empty.
If your iOS app requests user location information, you can skip this step.
- If the iOS app does not have permission to save data on the mobile device, add Privacy - Photo Library Usage Description and Privacy - Photo Library Additions Usage Description keys with values to the Info.plist file. The values specified are shown to the customer in the permission request message.
Importing libraries in Objective-C
To add a library into your iOS app:
- Copy the
ecommpaySDK.xcframework
file in the project folder of you iOS app. - Add the library into your project. When using Xcode 12, you need to do the following:
- Open the target of your project.
- Select .
- Click +.
- Click Add Other.
- Select the
ecommpaySDK.xcframework
file and click Add. - Select Build Settings.
- Set Always embed swift embedded libraries to Yes.
- Add key NSCameraUsageDescription with value
permission is needed in order to scan card
to the Info.plist file. - If your iOS app does not use user location information, add the NSLocationWhenInUseUsageDescription key with the
fraud prevention
value in the Info.plist file.The ecommpay libraries code does not request user location if the request is not initiated by the host app, but the App Store requires that the NSLocationWhenInUseUsageDescription key value is not empty.
If your iOS app requests user location information, you can skip this step.
- If the iOS app does not have permission to save data on the mobile device, add Privacy - Photo Library Usage Description and Privacy - Photo Library Additions Usage Description keys with values to the Info.plist file. The values specified are shown to the customer in the permission request message.
Importing libraries via Cocoapods
To add a library into your iOS app:
- Open the Podfile file and add the following strings:
target 'App' do # Pods for App pod 'EcommpaySDK_UI' end
- Add key NSCameraUsageDescription with value
permission is needed in order to scan card
to the Info.plist file. - If your iOS app does not use user location information, add the NSLocationWhenInUseUsageDescription key with the
fraud prevention
value in the Info.plist file.The ecommpay libraries code does not request user location if the request is not initiated by the host app, but the App Store requires that the NSLocationWhenInUseUsageDescription key value is not empty.
If your iOS app requests user location information, you can skip this step.
- If the iOS app does not have permission to save data on the mobile device, add Privacy - Photo Library Usage Description and Privacy - Photo Library Additions Usage Description keys with values to the Info.plist file. The values specified are shown to the customer in the permission request message.
Signature generation
Make sure that the data is signed on the server side of the web service with the use of the secret key received from ecommpay. To work with the signature, you can use ready-to-use components, such as language-specific SDKs for web services (details), or your own in-house solutions. The procedure of working with the signature is described in Signature generation and verification.
Testing
If necessary, you can open the payment form in the test mode in order to get information about errors if there were any when payment parameters were specified or to test processing payments with a certain payment result. When creating the request to open the payment form, in the PaymentOptions
object specify the following values for the mockModeType
parameter (the values are listed for Swift and Objective-C respectively):
MockModeType.success
/MockModeTypeSuccess
—if you need to receiveSuccess
payment result.MockModeType.decline
/MockModeTypeDecline
—if you need to receiveDecline
payment result.
If you need to switch to the production mode, pass MockModeType.disabled
/ MockModeTypeDisabled
as a value for the mockModeType
parameter.
You can also test payment processing in the test environment of the ecommpay payment platform. In this case, you should get access to the ecommpay test environment (it can be done via an application on the company's main site) and use the identifier and the secret key of the test project as values of the required parameters passed in the request to open the payment form.
The code samples that are provided on Github contain constants for these parameters
let secret = "your_secret" // secret key of the test project let project_id: Int32 = 10 // identifier of the test project
#define SECRET @"your_secret" // secret key of the test project #define PROJECT_ID 10 // identifier of the test project
To switch to the production mode, change the test values (the identifier and the secret key of the test project) to the production ones.
Use
Opening payment form
SDK UI & Core for iOS supports such actions as performing one-time purchases and placing authorisation holds as part of executing two-step purchases, registering COF purchases and performing payment card verification. To initiate these actions, you need a certain parameter set. The required minimum of parameters is passed in the PaymentOptions
object. Optional parameters can be passed in the same object. In addition, they can also be requested from the customer or received from the payment platform.
Opening the payment form in Swift
To open the payment form:
- Import the library:
import ecommpaySDK
- Declare the EcommpaySDK library in you app (for example, inside the
viewDidLoad
method):let ecommpaySDK = EcommpaySDK()
- Create an object named
PaymentOptions
. This object must contain the following required parameters:projectId
(integer)—a project identifier assigned by ecommpaypaymentId
(string)—a payment identifier unique within the projectpaymentCurrency
(string)—the payment currency code in the ISO 4217 alpha-3 formatpaymentAmount
(integer)—the payment amount in the smallest currency unitcustomerId
(string)—a customer's identifier within the project
For card payments, also pass the
additionalFields
parameter with at least one of the following parameters:customer_email
orcustomer_phone
.To specify the action you need, indicate the required operation type:
Sale
,Auth
, orVerify
in theaction
parameter. You can also add any other parameters listed in the following section.The following is an example of the
PaymentOptions
object that includes optional parameters (description of the payment and the customer's country)let paymentOptions = PaymentOptions(projectID: 10, paymentID: "internal_payment_id_1", paymentAmount: 1999, paymentCurrency: "USD", paymentDescription: "T-shirt with dog print", customerID: "10", regionCode: "US")
- Pack all payment parameters into a string for signing:
paymentOptions.paramsForSignature();
- Send the string to the server side of your web service.
- Have your web service generate the signature on the basis of the string and your secret key.
- Add signature in your
PaymentOptions
object:paymentOptions.signature = signature;
- Open the payment form by using the following code:
ecommpaySDK.presentPayment(at: self, paymentOptions: paymentOptions) { result in print("ecommpaySDK finished with status \(result.status.rawValue)") ... }
Before opening the payment form, the library checks for any errors and opens the payment form only if no errors occur. Otherwise, the payment form is not opened and the
presentPayment
method returns the error code.
Opening the payment form in Objective-C
To open the payment form:
- Import the library:
#import <EcommpaySDK/EcommpaySDK.h>
- Declare the EcommpaySDK library in you app (for example, inside the
viewDidLoad
method):EcommpaySDK *self.EcommpaySDK = [[EcommpaySDK alloc] init];
- Create an object named
PaymentOptions
. This object must contain the following required parameters:projectId
(integer)—a project identifier assigned by ecommpaypaymentId
(string)—a payment identifier unique within the projectpaymentCurrency
(string)—the payment currency code in the ISO 4217 alpha-3 formatpaymentAmount
(integer)—the payment amount in the smallest currency unitcustomerId
(string)—a customer's identifier within the project
For card payments, also pass the
additionalFields
parameter with at least one of the following parameters:customer_email
orcustomer_phone
.To specify the action you need, indicate the required operation type:
Sale
,Auth
, orVerify
in theaction
parameter. You can also add any other parameters listed in the following section.The following is an example of the
PaymentOptions
object that includes optional parameters (description of the payment and the customer's country)PaymentOptions *paymentOptions = [[PaymentOptions alloc] initWithProjectID:10 paymentID:@"internal_payment_id_1" paymentAmount:1999 paymentCurrency:@"USD" paymentDescription:@"T-shirt with dog print" customerID:@"10" regionCode:@"US"];
- Pack all payment parameters into a string for signing:
paymentOptions.paramsForSignature();
- Send the string to the server side of your web service.
- Have your web service generate the signature on the basis of the string and your secret key.
- Add signature in your
PaymentOptions
object:[paymentOptions setSignature:signature]
- Open the payment form by using the following code:
[self.EcommpaySDK presentPaymentAt:self paymentOptions:paymentOptions completionHandler:^(PaymentResult *result) { NSLog(@"EcommpaySDK finished with status %ld", (long)result.status); ... }];
Before opening the payment form, the library checks for any errors and opens the payment form only if no errors occur. Otherwise, the payment form is not opened and the
presentPayment
method returns the error code.
Processing payments
By default, SDK UI & Core for iOS allows processing one-step purchases (action type Sale
). This type of checkout works right out-of-the-box and requires no additional setup.
In addition, SDK UI & Core for iOS supports processing two-step purchases (which involves placing an authorisation hold via the SDK and subsequent debiting of the authorised amount). To perform a two-step purchase:
- Open the payment form with
Auth
specified as a value for the action type parameter in thepaymentOptions
object:Figure 6. Swift paymentOptions.action = .Auth
Figure 7. Objective-C [paymentOptions setAction: ActionTypeAuth];
- When needed, initiate debiting of the authorised amount via Dashboard (details) or Gate (by sending the request to the /v2/payment/card/capture endpoint).
Payment card verification
Payment instrument verification can be used when you need to validate a card without withdrawing funds instantly (for example, before performing a payout) or when you need to save card details for subsequent use. It is essentially a payment that involves debiting a dummy (zero) amount from the customer's card.
To perform verification, open the payment form with Verify
specified as a value for the action type parameter in the paymentOptions
object:
paymentOptions.action = .Verify
[paymentOptions setAction: ActionTypeVerify];
Payment status information
To receive payment result notifications, use requests with the following code included:
ecommpaySDK.presentPayment(at: self, paymentOptions: paymentOptions) { result in print("ecommpaySDK finished with status \(result.status.rawValue)") if let error = result.error { // if an error occurred print("ErrorCode: \(error.code) message: \(error.message)") } }
[self.ecommpaySDK presentPaymentAt:self paymentOptions:paymentOptions completionHandler:^(PaymentResult *result) { NSLog(@"EcommpaySDK finished with status %ld", (long)result.status); if(result.error != NULL) { // if an error occurred NSLog(@"Error code: %@ with message: %@", error.codeString, error.message); } }];
Possible payment result codes passed in the PaymentResult.status
parameter:
Result code | Message | Description |
---|---|---|
0 |
Success | Payment has been completed |
100 |
Decline | Payment has been declined |
200 |
Cancelled | Payment has been cancelled by the customer |
500 |
Error | An error occurred when the payment was being processed |
Processing payments made with Apple Pay
In order to implement payment processing which involves the Apple Pay payment method, it is necessary to do the following:
- Register the merchant's identifier (Merchant ID) with Apple. Merchant ID allows the merchant to accept payments made with the Apple Pay method. This identifier never expires and can be used in multiple websites and iOS applications. For more information see Apple documentation: Create a merchant identifier.
- Create Payment Processing Certificate. This certificate is associated with the Merchant ID and is used to secure transaction data when processing Apple Pay payments. For more information see Apple documentation: Create a payment processing certificate.
- Send Payment Processing Certificate to the ecommpay technical support. Use the agreed upon security methods.
- Enable the Apple Pay capability for the mobile application in the programming environment. For information about enabling Apple Pay capability in Xcode environment, see Apple documentation: Enable Apple Pay
Once these steps are completed, you can process Apple Pay payments. The main steps such as opening the payment form and processing the responses are performed according to the general procedure, which is the same for all payment methods. In addition, you need to pass the following data in the applePayOptions
object
setupApplePayparams(paymentOptions: PaymentOptions) { let applePayOptions = PaymentOptions.ApplePayOptions(applePayMerchantID: "merchant.example.com", applePayDescription: "Shop", countryCode: "US") paymentOptions.applePayOptions = applePayOptions }
setApplePaySettings:(PaymentOptions *)paymentOptions { PaymentOptionsForApplePay *applePayOptions = [[PaymentOptionsForApplePay alloc] initWithApplePayMerchantID:@"merchant.example.com" applePayDescription:@"Shop" countryCode:@"US"]; }
All parameters passed in the applePayOptions
object are mandatory and are necessary for the Apple Pay session to start correctly.
Additional capabilities
Submitting additional payment information
Generally, for processing a payment, it is enough to send a set of parameters that are mandatory for its initiation. However, in some cases, a payment system or a provider can require additional data necessary for processing a particular payment. This can be due to region-specific requirements, the need for an additional anti-fraud check, or other factors. The information about submitting additional payment data is provided in the following article.
The final set of required parameters can vary depending on a specific provider or a payment system. The list of parameters relevant for a particular payment is displayed to the customer on the payment form. The customer fills in the required data, confirms the payment, and receives the payment result information.
Cascade payment processing
In case of a payment attempt failure, the capability of cascade payment processing can be used (details). This capability implies executing a sequence of payment attempts via alternative providers without the payment method change and can be set up upon coordination with the ecommpay specialists.
If this capability is set up for the project in use, then after the first unsuccessful attempt, a notification is received from SDK UI & Core for iOS. This notification contains the cascading_with_redirect = true
attribute-value pair. Along with that, the error page with the button to retry making the payment is shown to the customer. If the 3‑D Secure authentication is not required as part of the additional attempt, then the attempt is executed without any further interaction with the customer. Otherwise, a separate page opens for repeating the authentication process.
Collecting customer data
In some cases, alongside the mandatory parameters, it can be relevant to require the additional ones (such as phone numbers and email addresses) from the customers. To have this capability set up, the merchant should decide which data has to be mandatory to be specified by the customers and communicate data collection preferences to the technical support specialists. For more information about using the capability, see the separate article.
Payment interface language support
By default, during the work with SDK UI & Core, the payment interface is localised according either to the language of the customer's device—if this language is supported for the project in use—or to a language set as default for other cases (generally, English). Along with that, if relevant, you can localise the payment interface for particular sessions. For this, every request for opening the payment form must contain a corresponding language code in the languageCode
(details).
The following languages are supported for the SDK interface and can be promptly set up in the projects of the payment platform.
Language | Code |
---|---|
English | en |
German | de |
Hungarian | hu |
Italian | it |
Spanish | es |
French | fr |
Saving payment data
SDK UI & Core for iOS allows saving payment data of the customer for subsequent processing of payments without the need for the said customer to re-enter such data. This capability is set up individually for each project. The merchant has to let the technical support know which of the two options is preferable: always save payment data or ask the customer to select the option. For more information about this capability, refer to article Saving customer payment data.
As a result of saving payment data, a separate identifier is generated for each payment instrument. This identifier is associated with the identifier of a certain customer (customerId
). To display saved payment data to the customer, pass false
in the hideSavedWallets
parameter of the PaymentOptions
object.
Payment form opening parameters
When processing card payments, pass the additionalFields
parameter in the PaymentOptions
object with at least one of the following fields.
Parameter | Description |
---|---|
|
Customer's email. Example: |
|
Customer's phone number. Example: |
In addition, when processing card payments, you are recommended to specify the customer's billing address information in the additionalFields
parameter.
Parameter | Description |
---|---|
|
Country of the customer's billing address in the ISO 3166-1 alpha-2 format (learn more). Example: |
|
City of the customer's billing address. Example: |
|
Postal code of the customer's billing address. Example: |
|
Street of the customer's billing address. Example: |
When working with SDK UI & Core for iOS, you can pass the following optional parameters in the PaymentOptions
object.
Parameter | Description |
---|---|
|
Description of the payment. A string that contains between 1 and 255 characters. Example: |
|
Data to be included in the notification with the list of the purchased items, passed as a JSON object encoded using the Base64 scheme. Example: |
|
Parameter to enable hiding or displaying saved payment instruments in the payment form. Possible values:
|
|
The identifier of the preselected payment method according to the table. Example: |
|
Object that contains additional objects and parameters necessary for the 3‑D Secure 2 authentication. |
|
Payment interface language code in the ISO 639-1 alpha-2 format. Must match one of the languages supported for the given project. Example: |
|
Code of the customer's country in the ISO 3166-1 alpha-2 format. Example: |
|
The Apple Pay identifier of the merchant. |
|
The description of the merchant in the Apple Pay service. |
|
Code of the customer's country in the ISO 3166-1 alpha-2 format. Passed when Apple Pay payments are processed. Example: |
|
A PNG or SVG file that contains the logo of the merchant. |
|
Payment interface colour passed as a Example: |
|
Additional fields that contain information about the customer. Includes a list of parameters with specified values. Example: |
To work with COF purchases, you should pass relevant parameters in the recurrentInfo
object of the PaymentOptions
object.
Parameter | Description |
---|---|
|
COF purchase type:
|
|
Debiting period for a regular purchase. Possible values:
|
|
Expiration day of the COF purchase. |
|
Expiration month of the COF purchase. |
|
Expiration year of the COF purchase. |
|
Identifier to assign to the COF purchase (for automatic debiting). Parameter must be passed together with the |
|
Date to perform the first debit in the dd-mm-yyyy format. Parameter must be passed together with the scheduledpaymentId parameter. |
|
Time when to perform a scheduled debit (for regular purchases) in the hh:mm:ss format, passed if the period parameter is specified as well. |
|
|
|
The amount to debit in the smallest currency unit. |
|
Date to perform the debit in the dd-mm-yyyy format. |
You can pass the following optional parameters in the threeDSecureInfo
object. Including these parameters increases the possibility of frictionless flow selection.
Parameter | Description |
---|---|
threeDSecureInfo —object of the ThreeDSecureInfo class containing additional objects and parameters used during the 3‑D Secure 2 authentication |
|
threeDSecurePaymentInfo —object of the ThreeDSecurePaymentInfo class with information about the purchase details and indication of the preferable authentication flow |
|
|
This parameter indicates whether challenge flow is requested for this payment. Possible values:
|
|
The dimensions of a window in which authentication page opens. Possible values:
|
|
The date the preordered merchandise will be available. Format: dd-mm-yyyy. |
|
This parameter indicates whether cardholder is placing an order for merchandise with a future availability or release date. Possible values:
|
|
This parameter indicates whether the cardholder is reordering previously purchased merchandise. Possible values:
|
threeDSecureGiftCardInfo —object of the ThreeDSecureGiftCardInfo class with information about payment with prepaid card or gift card. |
|
|
Amount of payment with prepaid or gift card denominated in the smallest currency unit. |
|
Currency of payment with prepaid or gift card in the ISO 4217 alpha-3 format, for example GBP. |
|
Total number of individual prepaid or gift cards/codes used in purchase. |
threeDSecureCustomerInfo —object of the ThreeDSecureCustomerInfo class with information about the customer. |
|
|
The parameter indicates whether the customer billing address matches the address specified in the Possible values:
|
|
State, province, or region code in the ISO 3166-2 format. Example: DOR for Dorset. |
|
Customer home phone number. Numeric, from 4 to 24 characters. Example: |
|
Customer work phone number. Numeric, from 4 to 24 characters. Example |
threeDSecureAccountInfo —object of the ThreeDSecureAccountInfo class with information about customer account details on record with the web service |
|
|
Additional customer account information, for instance arbitrary customer ID. Maximum 64 characters. |
|
Number of card payment attempts in the last 24 hours. Maximum 3 characters ( |
|
Number of card payment attempts in the last 365 days. Maximum 3 characters ( |
|
Number of days since the customer account was created. Possible values:
|
|
Any additional log in information in free text. Maximum 255 characters. |
|
Authentication type the customer used to log on to the account when placing the order. Possible values:
|
|
Account log on date and time. Format: dd-mm-yyyyhh:mm. |
|
Account creation date. Format: dd-mm-yyyy. |
|
Last account change date except for password change or password reset. Format: dd-mm-yyyy. |
|
Number of days since last customer account update, not including password change or reset. Possible values:
|
|
Last password change or password reset date. Format: dd-mm-yyyy. |
|
Number of days since the last password change or reset. Possible values:
|
|
Card record creation date. Format: dd-mm-yyyy. |
|
Number of days since the payment card details were saved in a customer account. Possible values:
|
|
Number of attempts to add card details in customer account in the last 24 hours. Maximum 3 characters ( |
|
Number of purchases with this cardholder account in the previous six months. Maximum 4 characters ( |
|
Suspicious activity detection result. Possible values:
|
threeDSecureShippingInfo —object of the ThreeDSecureShippingInfo class with shipping details. |
|
|
Shipping address. Maximum 150 characters. |
|
First shipping address usage date. Format: dd-mm-yyyy. |
|
Number of days since the first time usage of the shipping address. Possible values:
|
|
Shipping city. Maximum 50 characters. |
|
Shipping country in the ISO 3166-1 alpha-2 format, for example GB. |
|
The email for the digital content delivery. Maximum 255 characters. |
|
Shipment terms. Possible values:
|
|
Shipment recipient flag. Possible values:
|
|
Shipping postbox number. Maximum 16 characters. |
|
State, province, or region code in the ISO 3166-2 format. Example: If you specify this parameter, you need also to specify and populate the |
|
Shipment indicator. Possible values:
|
threeDSecureMpiResultInfo —object of the threeDSecureMpiResultInfo class with information about previous customer authentication |
|
|
The ID the issuer assigned to the previous customer operation. Maximum 36 characters. |
|
The flow the issuer used to authenticate the cardholder in the previous operation. Possible values:
|
|
Date and time of the previous successful customer authentication |